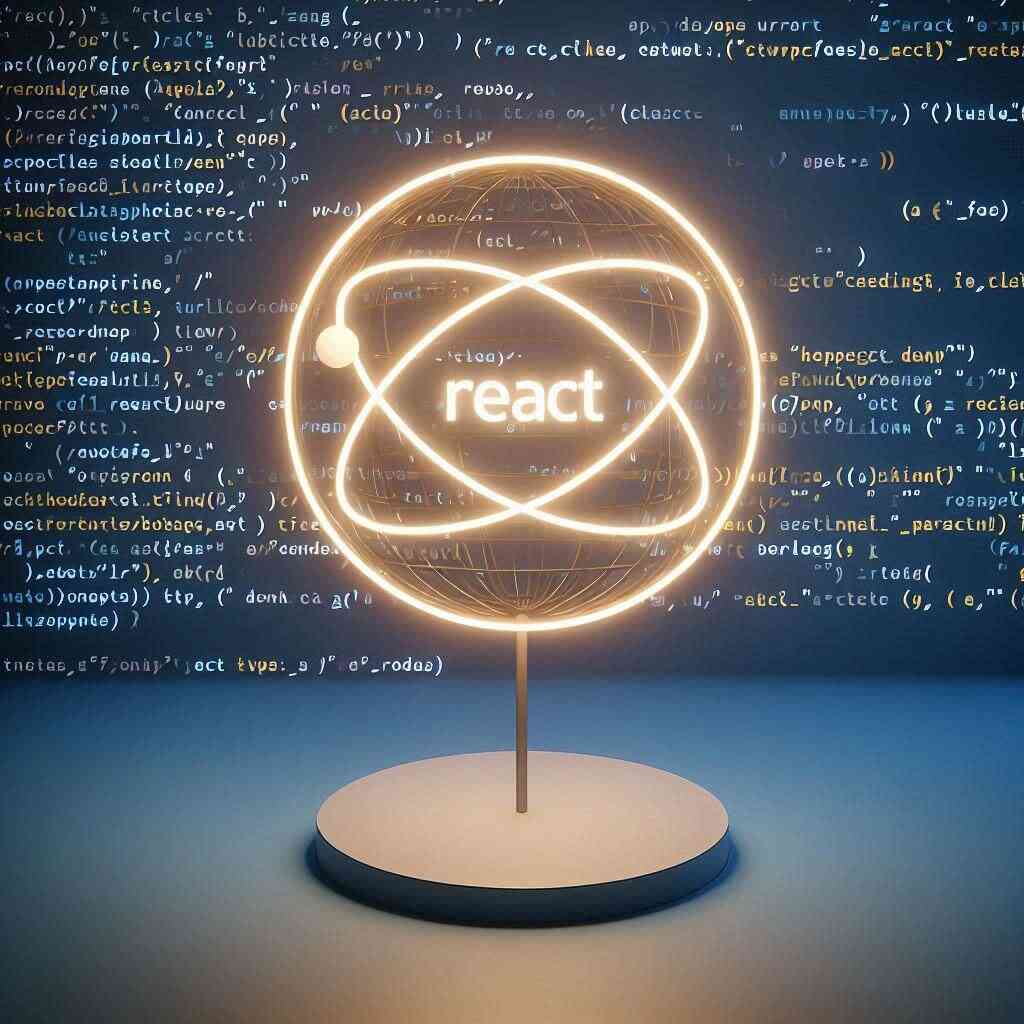
How to Create a React.js Project from Scratch?
Previously, we discussed 'What is React.js and why it is so popular,' and now, in this article, we will start from the ground up by creating new React apps. There are a few different approaches we can take, ranging from the simplest method using a CDN to the newest technique leveraging Vite. We'll explore each of these options, so you can choose the approach that best suits your needs and preferences when kickstarting your React.js projects.
Before starting you need to be sure that Node.js is installed in your local machine, if not you can use Node.js official documentation to pass this step. Okay, buckle up, and let's move.
1. Creating a New React.js App with CDN
We need to be clear that this method is not popular and usually useful for small apps. Nevertheless, it is really simple, so let's consider it.
- create a simple index.html file with a default template;
- inside the header tag we will import CDN scripts with "React", "React-Dom" and "babel";
- add an empty div in the body section with the "root" id (our app will be injected into this div);
- before closing the body tag we will add a script with React.js code (simple App function that returns JSX, and render function from ReactDom that will render our App function );
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>My React App</title>
<script src="https://unpkg.com/react@17/umd/react.production.min.js"></script>
<script src="https://unpkg.com/react-dom@17/umd/react-dom.production.min.js"></script>
<script src="https://unpkg.com/@babel/standalone/babel.min.js"></script>
</head>
<body>
<div id="root"></div>
<script type="text/babel">
function App() {
return (
<div>
<h1>Hello, React!</h1>
</div>
);
}
ReactDOM.render(<App />, document.getElementById('root'));
</script>
</body>
</html>
- save and open the index.html file in your browser, and you'll see "Hello, React!" header;
All the React code that we will paste into our App function will be executed and the result will be rendered on the browser page.
2. Setting Up a React Project with Create React App
Create React App is an officially supported tool that allows you to create a new React project with a pre-configured development environment, without having to spend time setting up build tools manually. It sets up a modern build pipeline and development server, allowing you to quickly start writing React code and seeing the changes live in your browser. Create React App comes with a set of sensible defaults and best practices to ensure your project is production-ready and optimized for performance right from the start. Sounds great, so let's check how we can use it.
- create a new folder "react-app" for example;
- open this folder with the help of VS Code or your favorite editor;
- open a new terminal window, and use the "npx create-react-app <app name>", we can use "." as an app name, and the new app will be created in the current folder;
That's all, yes so simple, after all, dependencies are installed, you can use the "npm run start" command to start the development server and build something incredible.
3. Introducing Vite: A Next-Gen React Project Starter
Vite is a modern and fast-build tool for web applications. It uses native ES modules to serve code, which means it doesn't need to bundle the entire app during development, making it incredibly fast to start and update. When starting a new React app, Vite is a great choice because it provides an extremely quick development server and lightning-fast hot module replacement, leading to a more efficient development experience. Additionally, Vite optimizes the build process for production, ensuring your final app is lean and performant.
- create a new folder "vite-react-app" for example;
- open this folder with the help of VS Code or your favorite editor;
- open a new terminal window, and use the "npm create vite@latest";
- answer a list of questions and select "project name" (set "." and the project will have the same name as the folder), "choose framework and variant";
- after the finish, package.json and starting files will be created with all default React.js settings, we need to use the "npm i" command, to install all the dependencies.
And the third project was created succesfully.
In this article, we've explored three distinct methods to kickstart your React.js projects: using a CDN for quick and simple setups, leveraging Create React App for a robust and pre-configured development environment, and adopting Vite for a next-gen, efficient build process. Each approach offers unique advantages, catering to different project needs and developer preferences.
By understanding and experimenting with these options, you can choose the one that best fits your workflow and project requirements. Now, you're equipped to start building amazing React applications with confidence. Happy coding!
Related
How to Add a QR Code Scanner in Vue.js (Step-by-Step Guide)
Starting out in programming is thrilling, yet the number of languages available makes it difficult to decide where to begin.
Building Simple CRM with Vue: Crafting Layouts and Navigation
Starting out in programming is thrilling, yet the number of languages available makes it difficult to decide where to begin.
Full-Stack Blogging CMS: A 17-Part Journey
Starting out in programming is thrilling, yet the number of languages available makes it difficult to decide where to begin.
Start the conversation